Sunday, January 12, 2025
Master Dynamic Programming: A Step-by-Step Guide
Posted by
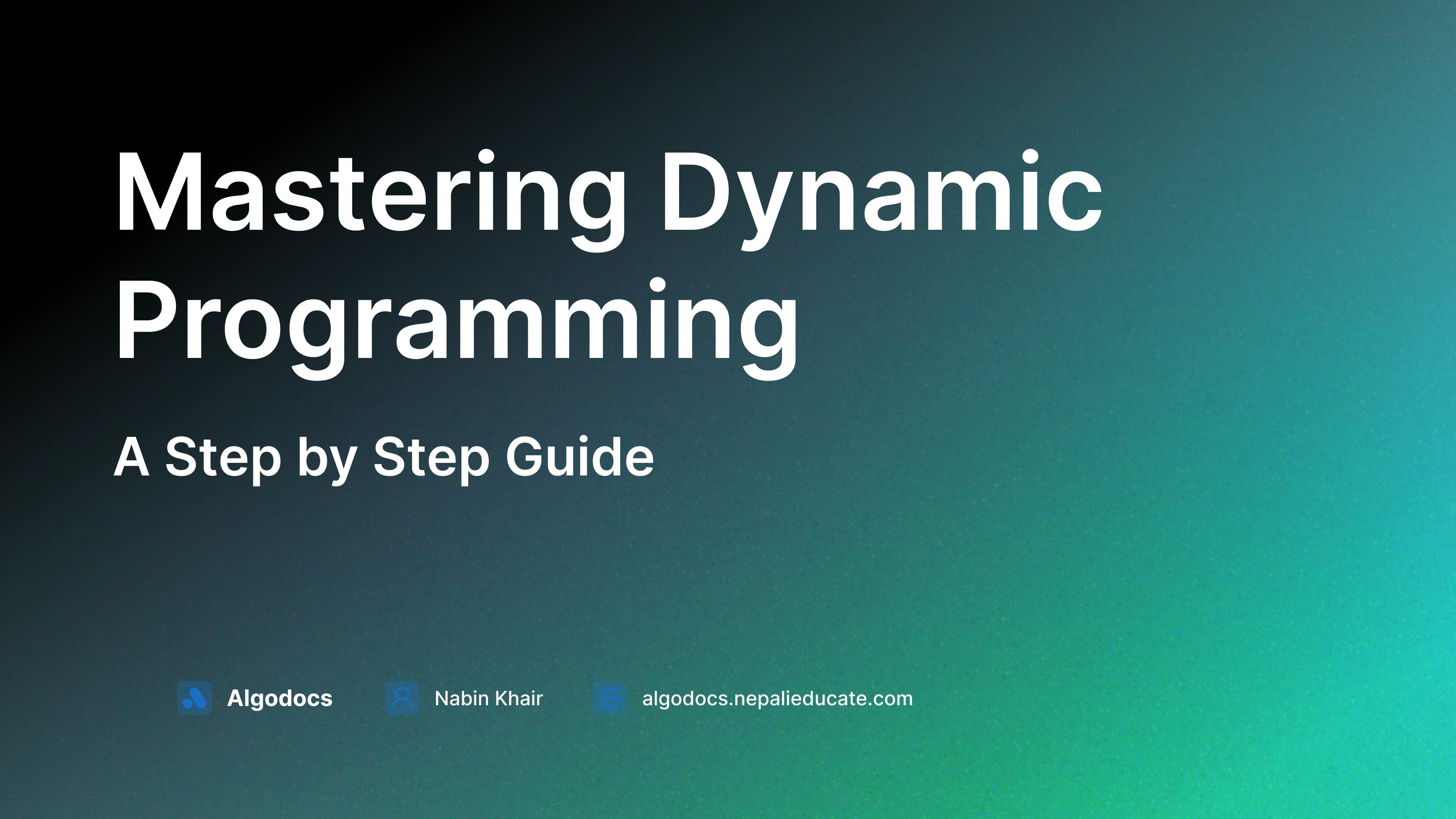
Introduction
Dynamic Programming (DP) is a powerful algorithmic paradigm that can solve complex problems by breaking them down into simpler subproblems. This guide will help you master DP concepts with practical examples.
Understanding the Basics
Dynamic Programming works on the principle of optimal substructure and overlapping subproblems. Let's break these down:
- Optimal Substructure: A problem has optimal substructure if its optimal solution can be constructed from optimal solutions of its subproblems.
- Overlapping Subproblems: When solving subproblems, the same subproblems are encountered multiple times.
Common DP Patterns
1. Fibonacci Sequence
The classic example to understand DP:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
2. The 0/1 Knapsack Problem
This is a fundamental DP problem that teaches important concepts:
def knapsack(values, weights, capacity):
n = len(values)
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(capacity + 1):
if weights[i-1] <= w:
dp[i][w] = max(
values[i-1] + dp[i-1][w-weights[i-1]],
dp[i-1][w]
)
else:
dp[i][w] = dp[i-1][w]
return dp[n][capacity]
Key Steps to Solve DP Problems
Identify if it's a DP problem
- Look for optimal substructure
- Check for overlapping subproblems
- See if you need to find an optimal value
Define the state
- What information do you need to represent a subproblem?
- What variables are changing in the recursive formula?
Write the recurrence relation
- How do smaller subproblems contribute to the larger problem?
- What decisions can you make at each step?
Decide the base cases
- What are the simplest instances of the problem?
- What values do you know without calculation?
Practice Problems
Here are some problems to practice, arranged by difficulty:
Easy
- Climbing Stairs
- Maximum Subarray
- House Robber
Medium
- Longest Increasing Subsequence
- Coin Change
- Unique Paths
Hard
- Edit Distance
- Regular Expression Matching
- Burst Balloons
Conclusion
Dynamic Programming is a crucial skill for both competitive programming and technical interviews. Practice these patterns regularly, and you'll start recognizing DP opportunities in new problems.
Remember: The key to mastering DP is not memorizing solutions but understanding the thought process behind breaking down problems into simpler sub problems.
Personal Suggestion::
Try implementing the practice problems mentioned above and compare your solutions with others. The best way to learn DP is through consistent practice and analysis of different approaches.